How to add custom filter for Frontend Manager dashboard
Note: First we have to hook the field template in the filter form
Note: Frontend Manager Filter has two action hooks. “wpcfe_before_shipment_filters” and “wpcfe_after_shipment_filters”. In this sample code will use the “wpcfe_after_shipment_filters” action hook.
Note: Frontend Manager Filter has two action hooks. “wpcfe_before_shipment_filters” and “wpcfe_after_shipment_filters”. In this sample code will use the “wpcfe_after_shipment_filters” action hook.
Copy and paste this code in you functions.php file in you active theme. Please follow the template structure so that it will not destroy filter form layout.
Using Dropdown Filter
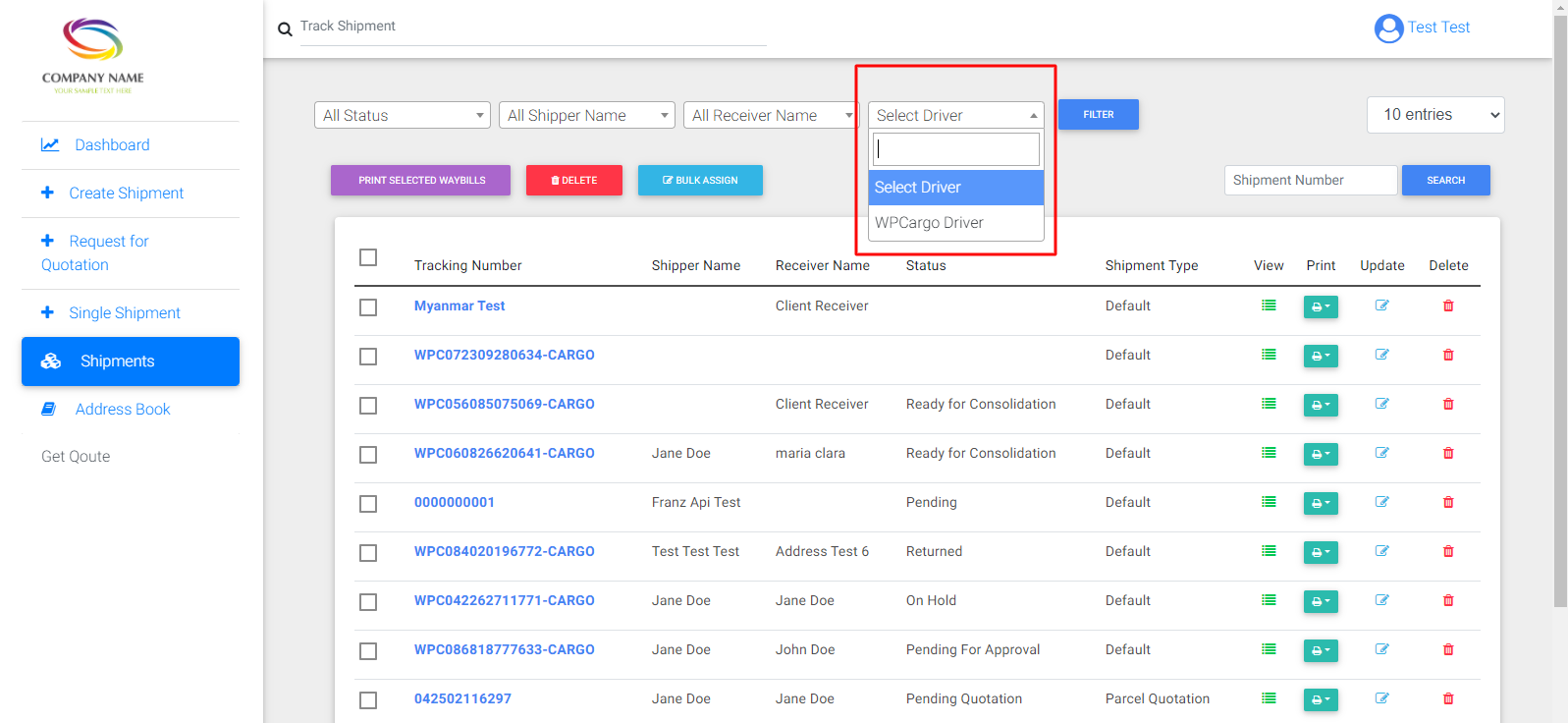
In this example we are using the WPCargo Driver List
function wpcfe_after_shipment_driver_filters_callback(){ $wpcargo_drivers = array(); if( function_exists( 'wpcargo_pod_get_drivers' ) ){ $wpcargo_drivers = wpcargo_pod_get_drivers(); } ?> <div class="form-group wpcfe-filter driver-filter p-0 mx-1"> <select id="driver-filter" name="wpcargo_driver" class="form-control md-form wpcfe-select"> <option value="">Select Driver</option> <?php foreach( $wpcargo_drivers as $driverID => $driver_name ): ?> <option value="<?php echo $driverID; ?>" <?php selected( get_post_meta( $shipment_id, 'wpcargo_driver', true ), $driverID ); ?>><?php echo $driver_name; ?></option> <?php endforeach; ?> </select> </div> <?php } add_action('wpcfe_after_shipment_filters', 'wpcfe_after_shipment_driver_filters_callback');
function wpcfe_dashboard_driver_meta_query_callback( $meta_query ){ /* * Note: $_GET['wpcargo_driver'] is the field name of our custom filter field * wpcargo_driveris the post meta key that we will search */ if( isset($_GET['wpcargo_driver']) && !empty( $_GET['wpcargo_driver'] ) ){ $meta_query[] = array( 'key' => 'wpcargo_driver', // shipment post meta key 'value' => urldecode( $_GET['wpcargo_driver'] ), // Form Submitted data 'compare' => 'LIKE' ); } return $meta_query; } add_filter( 'wpcfe_dashboard_meta_query', 'wpcfe_dashboard_driver_meta_query_callback', 10, 1 );
In this example we are using the Shipment Owner List
function wpcfe_after_shipment_onwner_filters_callback(){ $wpcargo_clients = get_users( array( 'role__in' =>; array( 'wpcargo_client' ) ) ); ?> <div class="form-group wpcfe-filter receiver-filter p-0 mx-1">; <label class="sr-only" for="registered_shipper">;<?php echo $receiver_data['label']; ?></label>; <select id="registered_shipper" name="registered_shipper" class="form-control md-form browser-default wpcfe-select">; <option value="">;Select Client</option>; <?php if( !empty( $wpcargo_clients ) ): > <?php foreach( $wpcargo_clients as $client ): ?> <option value="<?php echo $client->;ID; ?>">;<?php echo $client->;first_name.' '.$client->;last_name; ?></option>; <?php endforeach; ?> <?php endif; ?> </select>; </div>; <?php } add_action('wpcfe_after_shipment_filters', 'wpcfe_after_shipment_onwner_filters_callback');
function wpcfe_dashboard_owner_meta_query_callback( $meta_query ){ /* * Note: $_GET['registered_shipper'] is the field name of our custom filter field * registered_shipper is the post meta key that we will search */ if( isset($_GET['registered_shipper']) && !empty( $_GET['registered_shipper'] ) ){ $meta_query[] = array( 'key' => 'registered_shipper', // shipment post meta key 'value' => urldecode( $_GET['registered_shipper'] ), // Form Submitted data 'compare' => 'LIKE' ); } return $meta_query; } add_filter( 'wpcfe_dashboard_meta_query', 'wpcfe_dashboard_owner_meta_query_callback', 10, 1 );
In this example we are using the Branches List
function wpcfe_branch_after_shipment_filters_callback(){ $branches = array(); if( function_exists( 'wpcbm_get_all_branch' ) ){ $branches = wpcbm_get_all_branch(-1); } if( !empty( $branches ) ){ $assigned_branch = isset( $_GET['assigned_branch'] ) ? $_GET['assigned_branch'] : 0 ; ?>; <div class="form-group wpcfe-filter receiver-filter p-0 mx-1">; <label class="sr-only" for="assigned_branch">;Branch</label>; <select id="assigned_branch" name="assigned_branch" class="form-control md-form wpcfe-select">; <option value="">;<?php esc_html_e( 'Select Branch', 'wpcargo-branches' ); ?>;</option>; <?php foreach( $branches as $branch ) { ?>;<option value="<?php echo $branch->;id; ?>;" <?php selected( $assigned_branch, $branch->;id ); ?>;>;<?php echo $branch->;name; ?>;</option>;<?php } ?>; </select>; </div>; <?php } } add_action('wpcfe_after_shipment_filters', 'wpcfe_branch_after_shipment_filters_callback');
function wpcfe_branch_dashboard_meta_query_callback( $meta_query ){ /* * Note: $_GET['wpcargo_driver'] is the field name of our custom filter field * wpcargo_driveris the post meta key that we will search */ if( isset($_GET['assigned_branch']) && !empty( $_GET['assigned_branch'] ) ){ $meta_query[] = array( 'key' => 'shipment_branch', // shipment post meta key 'value' => urldecode( $_GET['assigned_branch'] ), // Form Submitted data 'compare' => 'LIKE' ); } return $meta_query; } add_filter( 'wpcfe_dashboard_meta_query', 'wpcfe_branch_dashboard_meta_query_callback', 10, 1 );
In this example we are using the Container Title
//Aditional filter for container number function wpcfe_after_shipment_driver_filters_callback(){ $wpcargo_drivers = array(); if( function_exists( 'wpcargo_pod_get_drivers' ) ){ $containers = wpccustom_get_all_containers(); } ?> <div class="form-group wpcfe-filter driver-filter p-0 mx-1"> <select id="container-filter" name="wpcargo_container" class="form-control md-form wpcfe-select"> <option value="">Select Container</option> <?php foreach( $containers as $key =>$value ): ?> <option value="<?php echo $value['ID']; ?>" <?php selected( $value['ID'] ); ?>><?php echo get_the_title( $value['ID'] ); ?></option> <?php endforeach; ?> </select> </div> <?php } add_action('wpcfe_after_shipment_filters', 'wpcfe_after_shipment_driver_filters_callback'); function wpccustom_jpk_container_met_query_callback( $meta_query ){ /* * Note: $_GET['wpcargo_driver'] is the field name of our custom filter field * wpcargo_driveris the post meta key that we will search */ if( isset($_GET['wpcargo_container']) && !empty( $_GET['wpcargo_container'] ) ){ $meta_query[] = array( 'key' => 'shipment_container', // shipment post meta key 'value' => urldecode( $_GET['wpcargo_container'] ), // Form Submitted data 'compare' => 'LIKE' ); } return $meta_query; } add_filter( 'wpcfe_dashboard_meta_query', 'wpccustom_jpk_container_met_query_callback', 10, 1 ); //function to fetch data from database function wpccustom_get_all_containers(){ global $wpdb; $sql = "SELECT `ID` FROM `{$wpdb->prefix}posts` WHERE `post_status` LIKE 'publish' AND `post_type` LIKE 'shipment_container'"; $result = $wpdb->get_results( $sql, ARRAY_A ); return $result; }